- typescript
- react
- node.js
- mysql
- html5
- css3
- gcp
Project background
StriveCloud, is white labelled software that adds game mechanics to any given app.
One of the popular game mechanics we implemented is a tier system. A famous example is booking.com. For every accomodation you book, you get points. With those points you can get into a higher tier. A higher tier means a bigger discount on future accomodations you book. People are incentivized to get into higher tiers, hence use the platform more, which is of course the end goal of any business. Our clients should be able to build their own tier system, however they want. That's where we started.
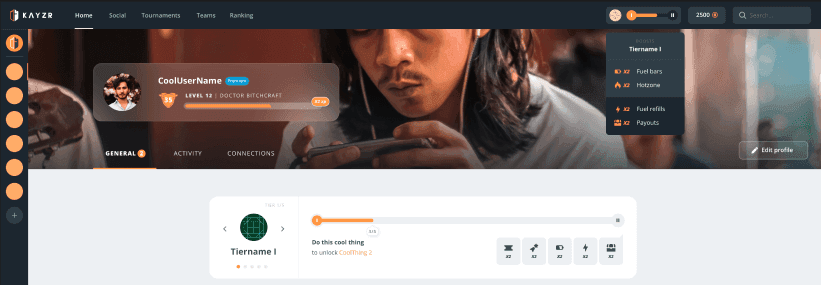
Approach
The process from idea to delivery of any feature would generally go like this:
- One or more clients requests a feature, which depending on the roadmap and the impact will get planned in accordingly. In this case, the tier system was an internal feature we wanted to develop for some time, and one we considered a high impact feature.
- Preparation is key! A small team gathers around the topic for a very first time, and roughly sketches the scope of how we'd want it to work; how we'd enable our clients to implement it; how their app users would interact with it. Everyone raises questions, and all this information is gathered in a doc any team member can access. It's one of the many features I took ownership of, so I started a technical analysis on the requirements, and presented a plan on how we could build it (required api's/database structure/data migration/frontend requirements/...).
- When most of the initial questions are resolved, the designer sketches a wireframe of how it could look, where all the important elements would be. This would be the base for another meeting, where we'd raise more questions. I'd keep on iterating over this process until there was a very clear understanding on how to build the feature, a clear understanding of what is in scope and what is out of scope.
- Once everything was defined, the final design was delivered. The product manager, designer and I perfected the UI & UX, and put the feature in a ready-to-start state.
- Time to get building! Creating features from A to Z is what I love most, and doing that in a timely manner is what I do best.
1import { RewardType } from '@shared/Enums';23const schema = {4tiers: { type: 'array', minItems: 2, items: { type: 'object', properties: {5 id: { type: 'objectid', nullable: true }, // only for update route6 name: { type: 'translations(255)' },7 description: { type: 'object', nullable: true, format: 'translations(255)' },8 icon: { type: 'uri' },9 threshold: { type: 'integer', minimum: 0 },10 rewards: { type: 'array', nullable: true, items: { type: 'object', properties: {11 type: { type: 'string', enum: [RewardType.SHOP_DISCOUNT, RewardType.CURRENCY_REGEN_SPEED, RewardType.XP_BOOST, ...] },12 currencyId: { type: 'objectid', nullable: true }, // required for [RewardType.SHOP_DISCOUNT, RewardType.CURRENCY_REGEN_SPEED]13 amount: { type: 'integer' },14 }}},15 notifications: { type: 'object', nullable: true, properties: {16 almostLostItNotification: { type: 'object', nullable: true, properties: {17 // to calc delta above threshold - if threshold is 100 and amount here is 10, as soon as points <= 110 user will get notification18 amount: { type: 'integer', minimum: 1 },19 title: { type: 'translations(255)' },20 description: { type: 'translations(255)' },21 }},22 almostMadeItNotification: { type: 'object', nullable: true, properties: {23 // to calc delta below reaching threshold - if threshold is 100 and amount here is 10, as soon as points >= 90 user will get notification24 amount: { type: 'integer', minimum: 1 },25 title: { type: 'translations(255)' },26 description: { type: 'translations(255)' },27 }},28 }}},29}},30...
Result
I delivered the feature spot on in the projected timeline and we released it behind feature flag for a couple of clients. After a period of testing, we removed the feature flag, and released it to all our clients - who were delighted to implement this new game mechanic. Below you can watch a little screencast of how our clients can create their own fully customizable tier system.
Some of the used skills
- technical analysis
- sprint planning
- ux/ui analysis
- api building
- form validation
- database migrations
- pixel perfect design implementations
- time management
- documenting